How and why you should update PHP to PHP 5.5 with Drupal
You should do this yesterday
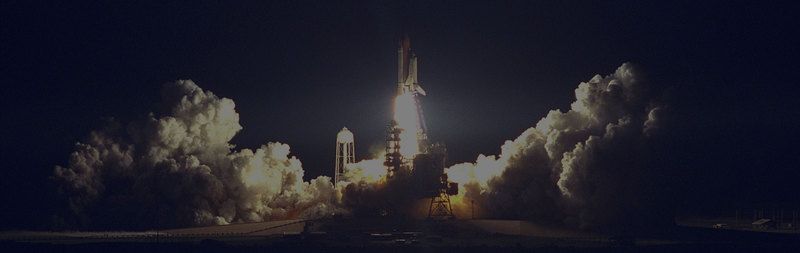
This post is a follow up to my previous blog post on how to upgrade PHP to 5.4 to support Drupal 8.
Why you should upgrade PHP
If you are looking for reasons to ditch PHP 5.3, here are some:
Security
PHP 5.3 reached end of life in August 2014, this means that if you are running this version, you are running an insecure version of PHP that potentially has security holes in it. This is bad for obvious reasons.
Bundled opcode cache
PHP 5.5 is the first version that bundles an opcode cache with PHP, this means there is also no need to also run APC (unless you need userland caching in APCu).
Performance
PHP profiled the 5.4 release compared to 5.3 for Drupal, and that found that:
- 7% more requests/second
- 50% PHP memory reduction
PHP 5.5 offers more performance again, and there is a section at the bottom of this article that goes through a real life scenario.
Cool new features
Read through the list of new features, here are some neat things you are missing out on:
- try/catch/finally is finally now in
- Short array syntax
$array = [
"foo" => "bar",
"bar" => "foo",
];
- Function array dereferencing
$secondElement = getArray()[1];
How to upgrade to PHP 5.5
There are a number of ways to update your server to PHP 5.5.
Upgrade to Ubuntu Trusty Tahr 14.04
Ubuntu Trusty Tahr 14.04 (which is an LTS version), which comes bundled with PHP 5.5.9. This is probably the best solution if you are managing your own Ubuntu box.
Install a PPA on Ubuntu Precise 12.04
If you are running the older Ubuntu Precise 12.04, you can add a PPA
sudo add-apt-repository ppa:ondrej/php5
sudo apt-get update
sudo apt-get install php5
php5 -v
It would be worth considering a dist upgrade though, but this at least can buy you some time.
Acquia Cloud UI
If you use Acquia Cloud for hosting there is a convenient PHP version selector in the UI.

More information can be found in the documentation. Be aware, once you upgrade beyond PHP 5.3, you cannot downgrade, so ensure you test your code on a development server first ;)
Common coding issues
Although Drupal 7 core, and most popular contributed modules will already support PHP 5.5, it would pay to do a code audit on any custom code written to ensure you are not using things you should not be. Here are some links you should read:
Below are some of the most common issues I have found in sites:
Call time pass-by-reference
If you have this in your code, you will have a bad time, as this is now a PHP fatal.
foo(&$a); // Bad times.
Only variables can be passed by reference
This will cause PHP to throw notices.
$ php -a
Interactive shell
php > ini_set('error_reporting', E_ALL);
php > var_dump(reset(explode('|', 'Jim|Bob|Cat')));
PHP Strict Standards: Only variables should be passed by reference in php shell code on line 1
Strict Standards: Only variables should be passed by reference in php shell code on line 1
string(3) "Jim"
Where you will likely find this in Drupal in my experience is when manually rendering nodes:
This code works in PHP 5.3, but will throw notices in PHP 5.5:
$rendered = drupal_render(node_view(node_load(1), 'teaser'));
The fix is to simply use a temporary variable:
$view = node_view(node_load(1), 'teaser');
$rendered = drupal_render($view);
The reason being that drupal_render()
expects a variable to be passed in (as it is passed by reference).
How do you find coding issues
Enable the syslog module, and tail that in your development environment, hunt down and fix as many notices and warnings as possible. The more noisy your logs are, the harder it is to find actual issues in them. While you are at it, turn off the dblog module, this is only helpful if you do not have access to your syslog (as it is a performance issue to be continually writing to the database).
Real world performance comparison
This was taken from a recent site that underwent a PHP 5.3 to 5.5 upgrade. Here are 2 New Relic overviews, taken with identical performance tests run against the same codebase. The first image is taken with PHP 5.3 running:

You can see PHP time is around 260ms of the request.

With an upgrade to PHP 5.5, the time spent in PHP drops to around 130ms. So this is around a a 50% reduction in PHP time. This not only makes your application faster, but also it means you can serve more traffic from the same hardware.
Comments
If you have gone through a recent PHP upgrade, I would be interested to hear how you found it, and what performance gains you managed to achieve.